스프링 @Service 어노테이션
스프링 @Component 어노테이션. Spring Service 주석은 클래스에만 적용할 수 있습니다. 클래스를 서비스 공급자로 표시하는 데 사용됩니다.
스프링 @Service 어노테이션
Spring @Service 주석은 일부 비즈니스 기능을 제공하는 클래스와 함께 사용됩니다. 스프링 컨텍스트는 주석 기반 구성 및 클래스 경로 스캔이 사용될 때 이러한 클래스를 자동 감지합니다.
스프링 @Service 예제
Spring 서비스 클래스를 생성할 간단한 Spring 애플리케이션을 생성해 봅시다. Eclipse에서 간단한 maven 프로젝트를 만들고 다음 스프링 코어 종속성을 추가합니다.
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.6.RELEASE</version>
</dependency>
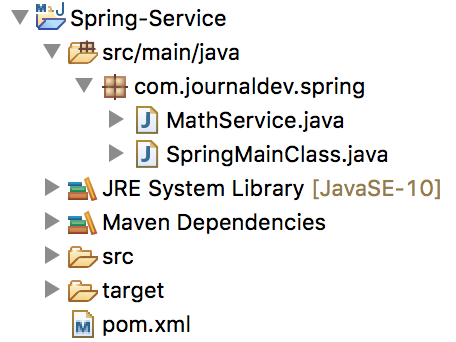
package com.journaldev.spring;
import org.springframework.stereotype.Service;
@Service("ms")
public class MathService {
public int add(int x, int y) {
return x + y;
}
public int subtract(int x, int y) {
return x - y;
}
}
두 정수를 더하고 빼는 기능을 제공하는 간단한 Java 클래스라는 점에 유의하십시오. 그래서 우리는 그것을 서비스 제공자라고 부를 수 있습니다. 스프링 컨텍스트가 자동 감지하고 컨텍스트에서 해당 인스턴스를 가져올 수 있도록 @Service 주석으로 주석을 달았습니다. 서비스 클래스의 인스턴스를 가져오는 주석 기반 스프링 컨텍스트를 생성할 기본 클래스를 생성해 봅시다.
package com.journaldev.spring;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class SpringMainClass {
public static void main(String[] args) {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext();
context.scan("com.journaldev.spring");
context.refresh();
MathService ms = context.getBean(MathService.class);
int add = ms.add(1, 2);
System.out.println("Addition of 1 and 2 = " + add);
int subtract = ms.subtract(2, 1);
System.out.println("Subtraction of 2 and 1 = " + subtract);
//close the spring context
context.close();
}
}
클래스를 Java 애플리케이션으로 실행하기만 하면 다음 출력이 생성됩니다.
Jun 05, 2018 3:02:05 PM org.springframework.context.support.AbstractApplicationContext prepareRefresh
INFO: Refreshing org.springframework.context.annotation.AnnotationConfigApplicationContext@ff5b51f: startup date [Tue Jun 05 15:02:05 IST 2018]; root of context hierarchy
Addition of 1 and 2 = 3
Subtraction of 2 and 1 = 1
Jun 05, 2018 3:02:05 PM org.springframework.context.support.AbstractApplicationContext doClose
INFO: Closing org.springframework.context.annotation.AnnotationConfigApplicationContext@ff5b51f: startup date [Tue Jun 05 15:02:05 IST 2018]; root of context hierarchy
MathService 클래스를 보면 서비스 이름을 "ms”로 정의했습니다. 이 이름을 사용하여 MathService
의 인스턴스도 가져올 수 있습니다. 이 경우 출력은 동일하게 유지됩니다. 그러나 우리는 명시적 캐스팅을 사용해야 합니다.
MathService ms = (MathService) context.getBean("ms");
여기까지가 Spring @Service 어노테이션의 간단한 예입니다.
GitHub 리포지토리에서 예제 프로젝트 코드를 다운로드할 수 있습니다.
참조: API 문서